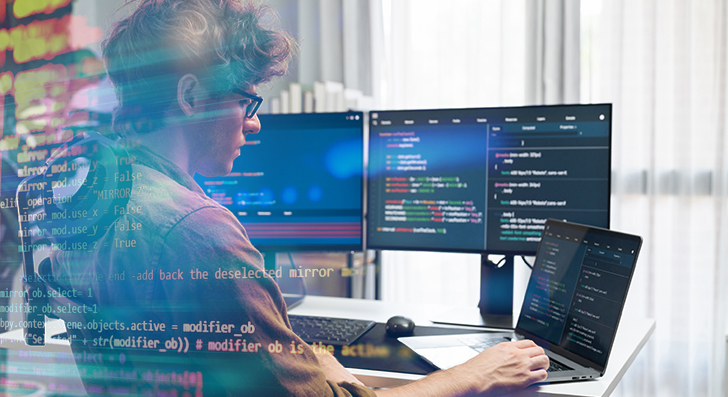
Scalability implies your software can take care of growth—extra people, a lot more information, and much more traffic—without the need of breaking. Being a developer, developing with scalability in your mind saves time and stress later on. Right here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not one thing you bolt on afterwards—it should be section of the plan from the beginning. Many apps are unsuccessful whenever they expand fast due to the fact the original layout can’t handle the additional load. As being a developer, you'll want to Believe early regarding how your program will behave stressed.
Begin by planning your architecture to be versatile. Stay clear of monolithic codebases exactly where anything is tightly related. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial sections. Each module or support can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle a million buyers or just a hundred? Choose the proper form—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t want them nevertheless.
A different significant issue is to avoid hardcoding assumptions. Don’t create code that only functions below existing problems. Give thought to what would happen if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use layout designs that assist scaling, like information queues or party-pushed devices. These support your app manage a lot more requests without having acquiring overloaded.
Whenever you Make with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing upcoming complications. A properly-planned system is easier to take care of, adapt, and improve. It’s greater to organize early than to rebuild later.
Use the ideal Databases
Selecting the correct databases is usually a critical Section of developing scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong one can slow you down or maybe bring about failures as your app grows.
Start by knowledge your info. Is it remarkably structured, like rows within a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient in shape. They are potent with associations, transactions, and regularity. Additionally they help scaling procedures like read through replicas, indexing, and partitioning to handle far more visitors and data.
If the information is a lot more flexible—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing huge volumes of unstructured or semi-structured details and may scale horizontally far more easily.
Also, take into account your browse and compose designs. Are you carrying out plenty of reads with less writes? Use caching and skim replicas. Are you handling a weighty generate load? Consider databases that could tackle high compose throughput, or even occasion-based mostly facts storage units like Apache Kafka (for temporary info streams).
It’s also smart to Feel forward. You might not will need Highly developed scaling functions now, but picking a databases that supports them suggests you received’t have to have to modify later.
Use indexing to speed up queries. Avoid pointless joins. Normalize or denormalize your info dependant upon your obtain styles. And normally monitor databases performance when you mature.
In short, the right databases relies on your application’s structure, speed needs, And exactly how you hope it to improve. Acquire time to choose properly—it’ll conserve plenty of difficulty later.
Optimize Code and Queries
Quick code is essential to scalability. As your application grows, just about every modest delay adds up. Poorly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s vital that you Make productive logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Alternative if an easy 1 is effective. Maintain your functions small, targeted, and easy to check. Use profiling tools to uncover bottlenecks—areas where your code takes far too extended to operate or makes use of too much memory.
Upcoming, take a look at your databases queries. These frequently gradual issues down much more than the code itself. Make sure Just about every query only asks for the information you truly want. Stay clear of Pick out *, which fetches every little thing, and in its place pick precise fields. Use indexes to speed up lookups. And keep away from accomplishing a lot of joins, especially across substantial tables.
In the event you observe a similar info staying asked for repeatedly, use caching. Keep the effects temporarily applying tools like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your database functions after you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra productive.
Make sure to take a look at with significant datasets. Code and queries that work fantastic with one hundred data could crash every time they have to take care of one million.
In short, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and a lot more website traffic. If anything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application rapid, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As opposed to a single server performing all the work, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for precisely the same info all over again—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You may serve it within the cache.
There are 2 common forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down database more info load, increases speed, and can make your application a lot more economical.
Use caching for things that don’t alter generally. And always be sure your cache is updated when info does improve.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app cope with more consumers, continue to be quickly, and Get better from problems. If you intend to improve, you need the two.
Use Cloud and Container Instruments
To make scalable applications, you will need instruments that permit your application grow very easily. That’s the place cloud platforms and containers are available. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential capability. When targeted traffic boosts, you may increase more resources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on setting up your application in place of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 device. This causes it to be straightforward to move your application amongst environments, out of your laptop into the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it instantly.
Containers also make it straightforward to independent aspects of your app into services. You can update or scale sections independently, which can be perfect for functionality and reliability.
In a nutshell, employing cloud and container tools signifies you are able to scale rapid, deploy effortlessly, and Get well rapidly when challenges occur. In order for you your app to increase without limitations, begin working with these tools early. They preserve time, cut down threat, and make it easier to stay focused on making, not fixing.
Check Anything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is executing, location problems early, and make greater conclusions as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just watch your servers—observe your app as well. Keep watch over just how long it requires for consumers to load web pages, how often problems take place, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a Restrict or simply a assistance goes down, it is best to get notified immediately. This allows you take care of difficulties rapidly, typically just before end users even recognize.
Monitoring is also practical any time you make alterations. Should you deploy a brand new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and facts maximize. With no monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal instruments in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about knowledge your program and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big providers. Even tiny applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you could Construct applications that grow easily without the need of breaking under pressure. Start off compact, Believe massive, and build wise.